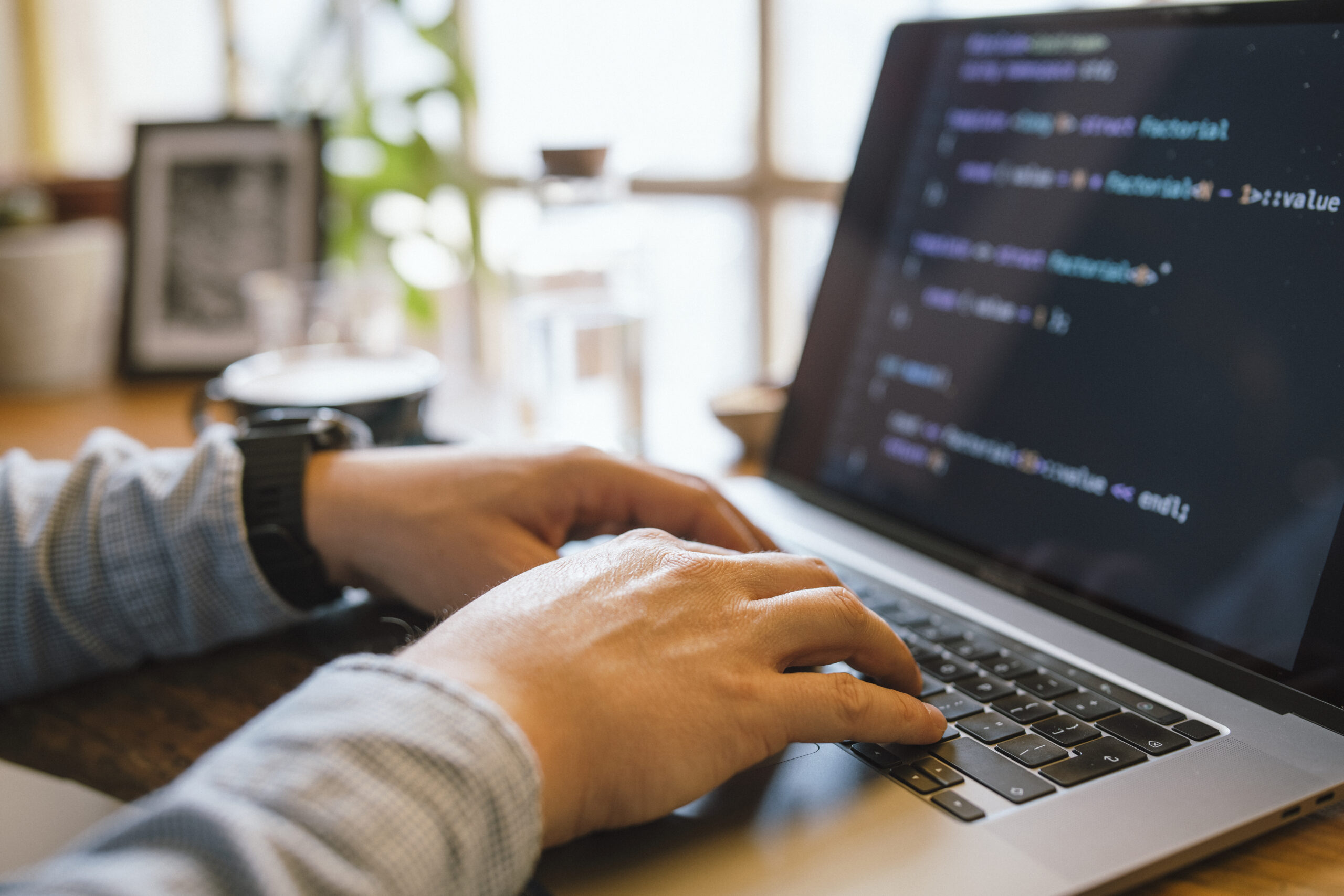
Debugging is The most critical — however typically forgotten — competencies in a developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and learning to think methodically to solve problems effectively. Regardless of whether you're a novice or possibly a seasoned developer, sharpening your debugging techniques can help save hrs of stress and substantially increase your productiveness. Allow me to share many techniques to aid developers level up their debugging activity by me, Gustavo Woltmann.
Learn Your Equipment
One of several quickest methods builders can elevate their debugging techniques is by mastering the equipment they use daily. Whilst writing code is a person Element of progress, being aware of the best way to interact with it correctly through execution is equally important. Modern-day growth environments come Geared up with effective debugging capabilities — but quite a few developers only scratch the area of what these equipment can do.
Acquire, as an example, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, action via code line by line, and perhaps modify code within the fly. When used correctly, they Enable you to observe just how your code behaves during execution, that's invaluable for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, keep an eye on community requests, see authentic-time overall performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can switch disheartening UI concerns into workable responsibilities.
For backend or method-degree builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate over working procedures and memory administration. Learning these equipment could possibly have a steeper Discovering curve but pays off when debugging efficiency troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, become cozy with Model Regulate units like Git to know code historical past, uncover the precise instant bugs were being introduced, and isolate problematic adjustments.
Eventually, mastering your instruments suggests likely beyond default settings and shortcuts — it’s about building an intimate familiarity with your progress ecosystem in order that when troubles occur, you’re not missing in the dead of night. The greater you are aware of your applications, the greater time you'll be able to devote fixing the actual difficulty as opposed to fumbling by means of the method.
Reproduce the trouble
One of the more important — and infrequently missed — techniques in productive debugging is reproducing the challenge. Ahead of jumping into the code or earning guesses, builders will need to make a constant environment or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug will become a match of opportunity, often bringing about wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as is possible. Request queries like: What actions brought about the issue? Which ecosystem was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it will become to isolate the exact ailments below which the bug takes place.
After you’ve gathered adequate information, try and recreate the issue in your neighborhood environment. This might mean inputting precisely the same data, simulating related user interactions, or mimicking technique states. If the issue appears intermittently, look at creating automatic exams that replicate the sting cases or state transitions included. These checks not just enable expose the problem but in addition reduce regressions Later on.
Often, The difficulty might be setting-unique — it might take place only on selected functioning methods, browsers, or beneath unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this kind of bugs.
Reproducing the trouble isn’t merely a action — it’s a mindset. It demands persistence, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you're already halfway to fixing it. With a reproducible scenario, You should use your debugging resources a lot more efficiently, examination likely fixes securely, and talk a lot more Obviously along with your group or consumers. It turns an abstract complaint into a concrete obstacle — Which’s where by builders prosper.
Browse and Have an understanding of the Mistake Messages
Mistake messages will often be the most valuable clues a developer has when one thing goes Improper. As opposed to observing them as discouraging interruptions, builders need to understand to treat mistake messages as direct communications in the method. They often show you just what exactly took place, in which it happened, and occasionally even why it happened — if you know the way to interpret them.
Commence by studying the information meticulously and in full. Quite a few developers, especially when less than time strain, glance at the 1st line and quickly begin earning assumptions. But deeper during the mistake stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like google — browse and realize them first.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it stage to a certain file and line quantity? What module or purpose triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also handy to know the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and learning to recognize these can considerably speed up your debugging approach.
Some faults are vague or generic, and in All those instances, it’s critical to look at the context by which the error happened. Verify linked log entries, enter values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings either. These typically precede larger sized problems and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a additional economical and self-assured developer.
Use Logging Wisely
Logging is Probably the most potent resources within a developer’s debugging toolkit. When utilised properly, it provides true-time insights into how an application behaves, aiding you recognize what’s taking place beneath the hood with no need to pause execution or phase throughout the code line by line.
An excellent logging method begins with understanding what to log and at what level. Common logging levels involve DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information and facts all through enhancement, Details for standard activities (like effective start-ups), Alert for probable troubles that don’t break the applying, Mistake for real issues, and Lethal if the program can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. Too much logging can obscure vital messages and decelerate your program. Focus on critical functions, state improvements, input/output values, and critical conclusion factors inside your code.
Structure your log messages clearly and consistently. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs let you keep track of how variables evolve, what problems are met, and what branches of logic are executed—all without having halting This system. They’re Specially valuable in generation environments exactly where stepping by code isn’t feasible.
On top of that, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. Which has a effectively-thought-out logging strategy, you can decrease the time it takes to spot difficulties, acquire further visibility into your purposes, and improve the Over-all maintainability and reliability of one's code.
Assume Similar to a Detective
Debugging is not just a specialized process—it is a type of investigation. To proficiently identify and resolve bugs, developers ought to solution the process like a detective fixing a thriller. This mentality helps break down elaborate issues into manageable elements and comply with clues logically to uncover the basis bring about.
Get started by accumulating proof. Look at the signs and symptoms of the trouble: error messages, incorrect output, or functionality difficulties. Identical to a detective surveys against the law scene, obtain just as much applicable information as you can with out jumping to conclusions. Use logs, test cases, and person experiences to piece alongside one another a transparent photo of what’s taking place.
Up coming, type hypotheses. Inquire your self: What might be causing this actions? Have any variations a short while ago been designed on the codebase? Has this challenge transpired just before below comparable circumstances? The intention will be to slim down choices and identify opportunity culprits.
Then, take a look at your theories systematically. Try to recreate the condition in a very controlled environment. For those who suspect a certain function or ingredient, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the outcomes guide you closer to the reality.
Pay out close consideration to little aspects. Bugs typically hide from the least predicted locations—similar to a missing semicolon, an off-by-just one error, or a race issue. Be thorough and client, resisting the urge to patch the issue devoid of totally knowledge it. Short-term fixes may perhaps conceal the actual issue, just for it to resurface afterwards.
Finally, retain notes on Everything you tried out and learned. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future issues and aid Many others comprehend your reasoning.
By imagining similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be more practical at uncovering hidden problems in intricate units.
Write Exams
Composing assessments is among the simplest methods to increase your debugging techniques and overall advancement effectiveness. Assessments not simply enable capture bugs early but will also serve as a security Web that gives you self-confidence when creating adjustments to the codebase. A properly-examined software is simpler to debug since it lets you pinpoint just the place and when a challenge happens.
Begin with unit exams, which give attention to personal functions or modules. These little, isolated tests can quickly reveal whether a selected bit of logic is Performing as predicted. Any time a exam fails, you promptly know wherever to glance, drastically minimizing time spent debugging. Device assessments are Specially beneficial for catching regression bugs—problems that reappear following Beforehand staying mounted.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These support make certain that numerous aspects of your software function alongside one another efficiently. They’re specifically useful for catching bugs that come about in sophisticated methods with various elements or services interacting. If a thing breaks, your exams can show you which Section of the pipeline unsuccessful and beneath what conditions.
Producing exams also forces you to definitely Believe critically regarding your code. To test a element correctly, you would like to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of knowing naturally qualified prospects to raised code construction and less bugs.
When debugging a difficulty, composing a failing exam that reproduces the bug could be a robust first step. After the take a look at fails consistently, it is possible to deal with fixing the bug and look at your exam pass when The problem is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In short, creating assessments turns debugging from the frustrating guessing sport into a structured and predictable course of action—helping you catch a lot more bugs, more rapidly plus much more reliably.
Take Breaks
When debugging a tricky problem, it’s effortless to be immersed in the situation—gazing your screen for hours, attempting Remedy soon after Option. But One of the more underrated debugging applications is simply stepping absent. Having breaks helps you reset your mind, decrease aggravation, and often see the issue from a new perspective.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You would possibly get started overlooking obvious errors or misreading code that you simply wrote just hours earlier. In this state, your brain becomes much less efficient at dilemma-fixing. A short walk, a espresso crack, or simply switching to a unique undertaking for ten–15 minutes can refresh your concentrate. Many builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially in the course of longer debugging classes. Sitting before a display screen, mentally stuck, is not only unproductive and also draining. Stepping away enables you to return with renewed energy in addition to a clearer way of thinking. You could suddenly see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
In the event you’re stuck, a very good guideline should be to set a timer—debug actively for forty five–60 minutes, then have a five–10 moment break. Use that point to move all around, extend, or do anything unrelated to code. It may well truly feel counterintuitive, especially beneath limited deadlines, nevertheless it basically results in a lot quicker and more effective debugging Eventually.
In short, using breaks will not be a sign of weak point—it’s a wise strategy. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel vision That always blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Each individual Bug
Each bug you come across is a lot more than simply A short lived setback—It is a chance to improve as a developer. Regardless of whether it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, each one can teach you one thing worthwhile for those who take the time to reflect and evaluate what went Mistaken.
Start out by inquiring you a few important concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater procedures like device screening, code evaluations, or logging? The solutions usually reveal blind spots inside your workflow or knowing and enable you to Construct more powerful coding routines shifting forward.
Documenting bugs can also be a wonderful pattern. Continue to keep a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and what you learned. Over time, you’ll begin to see designs—recurring concerns or typical mistakes—you could proactively stay clear of.
In staff environments, sharing Whatever you've discovered from the bug with the peers may be especially impressive. No matter if more info it’s by way of a Slack message, a brief create-up, or A fast expertise-sharing session, serving to Other folks avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll begin appreciating them as critical portions of your growth journey. In the end, a lot of the ideal developers are certainly not the ones who produce excellent code, but individuals that continually learn from their problems.
In the end, Every single bug you fix adds a completely new layer in your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Summary
Bettering your debugging techniques takes time, follow, and endurance — but the payoff is huge. It can make you a far more efficient, assured, and able developer. The subsequent time you might be knee-deep inside a mysterious bug, bear in mind: debugging isn’t a chore — it’s a possibility to be better at Everything you do.